SQLite是个抽象类,使用时需要创建一个自己的帮助类去继承它。
SQLiteOpenHelper中有2个抽象方法:onCreate()和 OnUpgrade()
SQLiteOpenHelper中有2个实例方法:getReadableDatabase() 和 getWritableDatabase()
SQLiteOpenHelper中有2个构造方法可重写,一般使用参数少一点的那个构造方法即可。
这个构造方法接收4个参数:
第1个:Context
第2个:数据库名
第3个:在查询数据的时候返回一个自定义的Cursor,一般都传入null
第4个:表示当前数据库的版本号,用于对数据库的升级操作
构建出SQLiteOpenHelpeer的实例后,再调用它的getReadDatabase()或getWritableDatabase()方法就能创建数据库了
创建数据库和升级数据库
XML:
1 2 3 4 5
| <Button android:id="@+id/create" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="create"/>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| package com.example.xxhuang.checkbox;
import android.content.Context; import android.database.sqlite.SQLiteDatabase; import android.database.sqlite.SQLiteOpenHelper; import android.os.Build; import android.widget.Toast;
public class MySQLite extends SQLiteOpenHelper {
public static final String CREATE_BOOK = "create table book (" + "id integer primary key autoincrement," + "author text," + "pages integer," + "name text)";
public static final String CREATE_CATEGORY = "create table category(" + "id integer primary key autoincrement," + "category_name text," + "category_code integory)";
private Context mContext;
public MySQLite(Context context, String name, SQLiteDatabase.CursorFactory factory, int version) { super(context, name, factory, version); mContext = context; }
@Override public void onCreate(SQLiteDatabase db) { db.execSQL(CREATE_BOOK); db.execSQL(CREATE_CATEGORY); Toast.makeText(mContext,"数据库创建成功",Toast.LENGTH_SHORT).show();
}
@Override public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) { db.execSQL("drop table if exists Book"); db.execSQL("drop table if exists Category"); onCreate(db); } }
|
对数据库进行增删改查:
SQLiteDatabase中提供了一些增删改查方法
添加:

三个参数:
表名 |
用于在未指定添加数据的情况下给某些可为空的列自动赋值NULL,一般不用到,直接传入null|ContentValues对象 |
它提供了一系列的put()方法重载,用于向ContentValues添加数据,只需要将表中的每个列名以及相应的待添加数据传入即可 |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| adddata按钮: adddata.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { SQLiteDatabase sqLiteDatabase = mySQLite.getWritableDatabase(); ContentValues values = new ContentValues();
values.put("name","3天精通java"); values.put("author","zhangsan"); values.put("page", 45); values.put("price", 12.34); sqLiteDatabase.insert("Book", null, values); values.clear();
values.put("name","3天精通Android"); values.put("author","lisi"); values.put("page", 20); values.put("price", 22.23); sqLiteDatabase.insert("Book",null,values);
} });
|
删除:

1 2 3 4 5 6 7
| deletedata 按钮
deletedata.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { SQLiteDatabase sqLiteDatabase = mySQLite.getWritableDatabase(); sqLiteDatabase.delete("Book","pages > ?",new String[]{"500"});
|
更新

分别是:表名、ContentValues、第三和第四个参数用来指定更新哪一行。
1 2 3 4 5 6 7 8 9 10 11
| updatedata按钮:
updatedata.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { SQLiteDatabase sqLiteDatabase = mySQLite.getWritableDatabase(); ContentValues contentValues =new ContentValues(); contentValues.put("price",12.00); sqLiteDatabase.update("Book", contentValues, "name = ?", new String[]{"3天精通java"}); } });
|
查询(查询还有很多方法):

参数:
- 表名
- 指定查询哪几列,不指定则默认查询所有列
- 用于约束查询某一行或几行的数据,不指定则默认查询所有行的数据
- 用于指定需要去group by的列,不指定则表示不对查询结果进行group by操作
- 用于对group by之后的数据进行进一步的过滤,不指定则表示不进行过滤
- 指定查询结果的排列方式
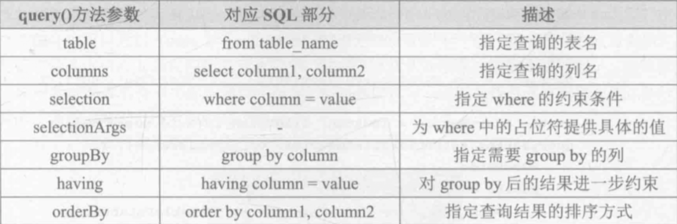
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| querydata按钮: querydata.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { SQLiteDatabase sqLiteDatabase = mySQLite.getWritableDatabase();
Cursor cursor = sqLiteDatabase.query("book",null,null,null,null,null,null);
if(cursor.moveToFirst()){ do { String name = cursor.getString(cursor.getColumnIndex("name")); int page = cursor.getInt(cursor.getColumnIndex("page")); double price = cursor.getDouble(cursor.getColumnIndex("price")); }while (cursor.moveToNext());
} cursor.close(); } });
|